My observation until now is that DataTables.js as a free JavaScript grid is an easier solution versus Ag-Grid. Later on I will try some enterprise features of Ag-Grid, maybe this will compensate for that.
Preliminary steps:
1. check the previous tutorial: https://mydev-journey.blogspot.com/2020/01/ag-grid-tutorial-with-aspnet-core-razor.html
1. application is live under: https://ag-grid2.zoltanhalasz.net/
2. zipped(cleaned) repo of the application: https://drive.google.com/open?id=10A0as_DTC94ve_oVtDF2uFW1cX19k4J7
How the app will look like:
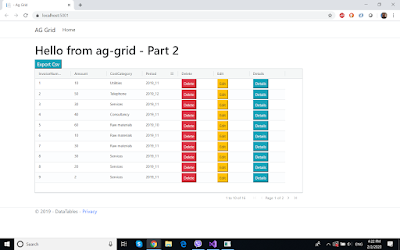
The steps of the tutorial are:
1. please remove the unnecessary files from the wwwroot (if you are using the part 1 repo).
2. check their official community resource from github, cloning their repo https://github.com/ag-grid/ag-grid/tree/master/community-modules/all-modules/dist
a. take the file ag-grid-community.min.js and copy it under wwwroot/js folder
(or ag-grid-community.noStyle.js)
b. the same, for the CSS files, taken from the Styles folder in the repo above
copy the them in the Css folder, and then reference them from the Layout file:
<link rel="stylesheet" href="~/css/ag-grid.css">
<link rel="stylesheet" href="~/css/ag-theme-balham.css">
3. the whole source of the index page will be:
<script src="~/js/ag-grid-community.min.js"></script>
<h1>Hello from ag-grid - Part 2</h1>
<div>
<div id="mybutton">
<button onclick="onExportCsv()" class="btn-info">Export Csv</button>
</div>
<div id="myGrid" style="height: 450px;width:900px;" class="ag-theme-balham"></div>
</div>
<script type="text/javascript" charset="utf-8">
var deleteRenderer = function(params) {
var eDiv = document.createElement('div');
eDiv.innerHTML = '<span class="my-css-class"><button class="btn-del btn-danger btn-xs">Delete</button></span>';
var eButton = eDiv.querySelectorAll('.btn-del')[0];
eButton.addEventListener('click', function() {
console.log('will be deleted, invoice no:', params.data.ID);
window.location.href = './Delete?id='+params.data.ID;
});
return eDiv;
}
var editRenderer = function (params) {
var eDiv = document.createElement('div');
eDiv.innerHTML = '<span class="my-css-class"><button class="btn-edit btn-warning btn-xs">Edit</button></span>';
var eButton = eDiv.querySelectorAll('.btn-edit')[0];
eButton.addEventListener('click', function() {
console.log('will be edited, invoice no:', params.data.ID);
window.location.href = './Edit?id='+params.data.ID;
});
return eDiv;
}
var detailsRenderer = function (params) {
var eDiv = document.createElement('div');
eDiv.innerHTML = '<span class="my-css-class"><button class="btn-details btn-info btn-xs">Details</button></span>';
var eButton = eDiv.querySelectorAll('.btn-details')[0];
eButton.addEventListener('click', function() {
console.log('will be info, invoice no:', params.data.ID);
window.location.href = './Detail?id='+params.data.ID;
});
return eDiv;
}
// specify the columns
var columnDefs = [
{ headerName: "InvoiceNumber", field: "InvoiceNumber" },
{ headerName: "Amount", field: "Amount" },
{ headerName: "CostCategory", field: "CostCategory" },
{ headerName: "Period", field: "Period" },
{ headerName: "Delete", field: null, cellRenderer: deleteRenderer },
{ headerName: "Edit", field: null, cellRenderer: editRenderer },
{ headerName: "Details", field: null, cellRenderer: detailsRenderer },
];
// let the grid know which columns to use
var gridOptions = {
columnDefs: columnDefs,
defaultColDef: {
sortable: true,
filter: true,
width: 120,
},
rowHeight : 35,
pagination: true,
paginationPageSize: 10,
};
// lookup the container we want the Grid to use
var eGridDiv = document.querySelector('#myGrid');
function getParams() {
return {
suppressQuotes: null,
columnSeparator: null,
customHeader: null,
customFooter: null
};
}
function onExportCsv() {
var params = getParams();
gridOptions.api.exportDataAsCsv(params);
}
// create the grid passing in the div to use together with the columns & data we want to use
new agGrid.Grid(eGridDiv, gridOptions);
agGrid.simpleHttpRequest({ url: './Index?handler=ArrayData' }).then(function (data) {
gridOptions.api.setRowData(data);
});
</script>
5. pagination
- this is solved using the gridOptions object, setting the properties:
pagination: true,
paginationPageSize: 10,
- this is solved using the gridOptions object, setting the properties:
pagination: true,
paginationPageSize: 10,
6. Csv export (basic)
- this is managed using the button with id = "mybutton", and writing its onclick event function:
function onExportCsv() {
var params = getParams();
gridOptions.api.exportDataAsCsv(params);
}
for the params object, I returned the basic features for a csv export using getParams function.
See more detailed explanation about csv export on their page: https://www.ag-grid.com/javascript-grid-csv/
- this is managed using the button with id = "mybutton", and writing its onclick event function:
function onExportCsv() {
var params = getParams();
gridOptions.api.exportDataAsCsv(params);
}
for the params object, I returned the basic features for a csv export using getParams function.
See more detailed explanation about csv export on their page: https://www.ag-grid.com/javascript-grid-csv/
7. height and width on the rows/columns
width: 120, // in defaultColDef properties
rowHeight: 35, // in gridOptions properties
width: 120, // in defaultColDef properties
rowHeight: 35, // in gridOptions properties
8. cell rendering (basic)
for example, in the columnDef for Delete
{ headerName: "Delete", field: null, cellRenderer: deleteRenderer },
deleteRenderer will be a function generating html for the delete button
var deleteRenderer = function(params) {
var eDiv = document.createElement('div');
eDiv.innerHTML = '<span class="my-css-class"><button class="btn-del btn-danger btn-xs">Delete</button></span>';
var eButton = eDiv.querySelectorAll('.btn-del')[0];
eButton.addEventListener('click', function() {
console.log('will be deleted, invoice no:', params.data.ID);
window.location.href = './Delete?id='+params.data.ID;
});
return eDiv;
}
for example, in the columnDef for Delete
{ headerName: "Delete", field: null, cellRenderer: deleteRenderer },
deleteRenderer will be a function generating html for the delete button
var deleteRenderer = function(params) {
var eDiv = document.createElement('div');
eDiv.innerHTML = '<span class="my-css-class"><button class="btn-del btn-danger btn-xs">Delete</button></span>';
var eButton = eDiv.querySelectorAll('.btn-del')[0];
eButton.addEventListener('click', function() {
console.log('will be deleted, invoice no:', params.data.ID);
window.location.href = './Delete?id='+params.data.ID;
});
return eDiv;
}
9. Detail, Edit, Delete Razor pages added for complete CRUD, as per cell rendering links suggest.
This is achieved using Entity Framework applied on the In-Memory database.
This is achieved using Entity Framework applied on the In-Memory database.
No comments:
Post a Comment